Several locales may be selected in the setup for multilingual users of Android 7.0 (API Level 24), which supports this feature. The budget for the locality object designates a particular geographic, political, or cultural region.
Locale-sensitive operations employ this locale to produce information for the user and are operations that need it to complete a job.
First step: Create a new project and resource files
In the first step to changing app language in Android, You need to start an Android Studio project from scratch.
We must construct a string resource file for the Gujarati language in this phase.
Right-click on App > Res > Values > Create > Value Resource File and give it the name strings.
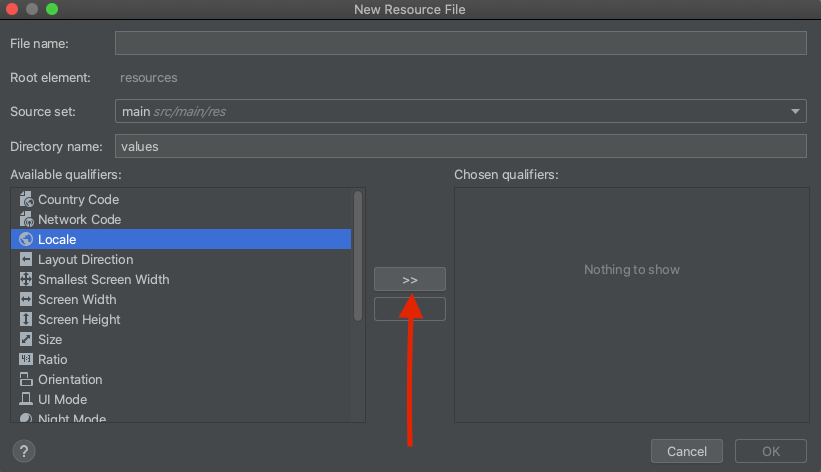
The next step is to choose Gujarati as the language and the qualifier as a locality from the drop-down menus, respectively. The stages are shown in the image below.
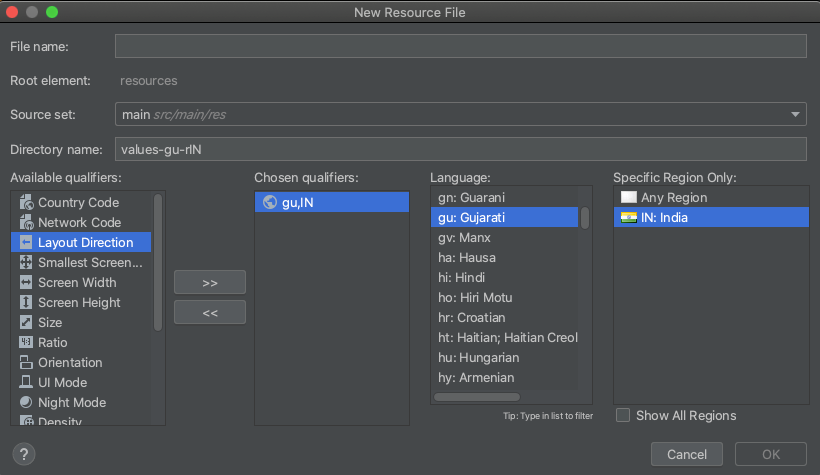
Now below is the resource file add strings.xml(gu-rlN) in the code and save the changes.
<resources>
<string name="app_name">Change Your App Language</string>
<string name="selected_language">ગુજરાતી</string>
<string name="language">કેમ છો?</string>
</resources>
And add this line to the string.xml file, which is the default for the English language.
<resources>
<string name="app_name">Change Your App Language</string>
<string name="selected_language">English</string>
<string name="language">How are you?</string>
</resources>
Step 2: Generate the layout file for the application
We’ll design the layout for our application in this phase. Navigate to apps> res> Layout> activity main.xml and add an image view for the drop_down icon along with two text views for the message and the selected language. The code sample for the activity_main.xml file is shown below.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="48dp"
android:text="Welcome To InfyOm"
android:textAlignment="center" />
<Button
android:id="@+id/btnGujarati"
android:layout_margin="16dp"
android:background="@color/colorPrimary"
android:textColor="#ffffff"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Gujarati"/>
<Button
android:id="@+id/btnEnglish"
android:layout_margin="16dp"
android:background="@color/colorPrimary"
android:textColor="#ffffff"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="English"/>
</LinearLayout>
Step 3: Build a LocaleHelper Class
Build a LocaleHelper Class in Step 3
We shall now develop a neighborhood assistance class. All of the functions necessary to alter the language in use are contained in this class. Create a new Java class with the name LocalHelper by selecting App > Java > Package from the context menu. The local helper class’s source code may be seen below.
import android.annotation.TargetApi;
import android.content.Context;
import android.content.SharedPreferences;
import android.content.res.Configuration;
import android.content.res.Resources;
import android.os.Build;
import android.preference.PreferenceManager;
import java.util.Locale;
public class LocaleHelper {
private static final String SELECTED_LANGUAGE=
"Locale.Helper.Selected.Language";
// the method is used to set the language at runtime
public static Context setLocale(Context context, String language) {
persist(context, language);
// updating the language for devices above android nougat
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
return updateResources(context, language);
}
// for devices having lower version of android os
return updateResourcesLegacy(context, language);
}
private static void persist(Context context, String language) {
SharedPreferences preferences=
PreferenceManager.getDefaultSharedPreferences(context);
SharedPreferences.Editor editor = preferences.edit();
editor.putString(SELECTED_LANGUAGE, language);
editor.apply();
}
// the method is used update the language of application by creating
// object of inbuilt Locale class and passing language argument to it
@TargetApi(Build.VERSION_CODES.N)
private static Context updateResources(Context context, String language) {
Locale locale = new Locale(language);
Locale.setDefault(locale);
Configuration configuration = context.getResources().getConfiguration();
configuration.setLocale(locale);
configuration.setLayoutDirection(locale);
return context.createConfigurationContext(configuration);
}
@SuppressWarnings("deprecation")
private static Context updateResourcesLegacy(Context context, String language)
{
Locale locale = new Locale(language);
Locale.setDefault(locale);
Resources resources = context.getResources();
Configuration configuration = resources.getConfiguration();
configuration.locale = locale;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR1) {
configuration.setLayoutDirection(locale);
}
resources.updateConfiguration(configuration,
resources.getDisplayMetrics());
return context;
}
}
Step 4: Working With the MainActivity.java File
This stage will include the use of Java code to switch between string.xml files in order to employ various languages. The LocalHelper class will be used to configure all the views and establish the click behavior on an Alert dialogue box so that the user may select their preferred language.
The code for the MainActivity.java class is shown below.
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.res.Resources;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
TextView messageView;
Button btnGujarati, btnEnglish;
Context context;
Resources resources;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
messageView = (TextView) findViewById(R.id.textView);
btnGujarati = findViewById(R.id.btnGujarati);
btnEnglish = findViewById(R.id.btnEnglish);
btnEnglish.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
context = LocaleHelper.setLocale(MainActivity.this, "en");
resources = context.getResources();
messageView.setText(resources.getString(R.string.language));
}
});
btnGujarati.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
context = LocaleHelper.setLocale(MainActivity.this, "hi");
resources = context.getResources();
messageView.setText(resources.getString(R.string.language));
}
});
}
}
Conclusion
So this was all about how you can programmatically change your app language in Android? We’ve given the complete source code of your problem, simply copy them and change accordingly.